ckeditor5:创建一个简单的插件
创建一个 insert-image 的插件
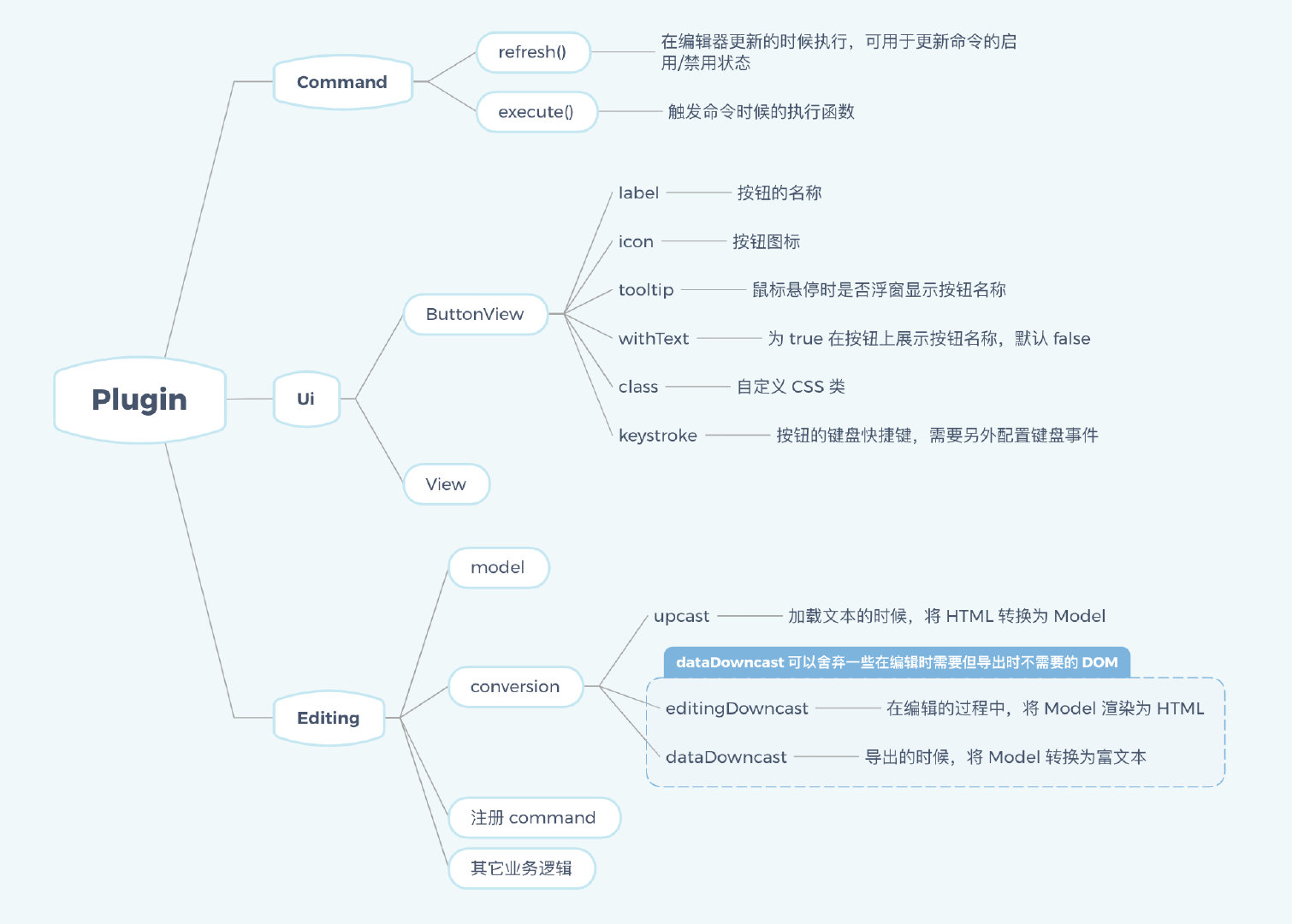
安装依赖
npm install --save @ckeditor/ckeditor5-image \
@ckeditor/ckeditor5-core \
@ckeditor/ckeditor5-ui
添加Image插件,保证图片能顺利显示
import Image from "@ckeditor/ckeditor5-image/src/image";
// ...
ClassicEditor.create(document.querySelector("#editor"), {
// Add Image to the plugin list.
plugins: [Essentials, Paragraph, Bold, Italic, Image],
// ...
});
// ...
HTML里放放测试图片
<div id="editor">
<p>Simple image:</p>
<figure class="image">
<img src="https://via.placeholder.com/1000x300/02c7cd/fff?text=Placeholder%20image" alt="CKEditor 5 rocks!">
</figure>
</div>
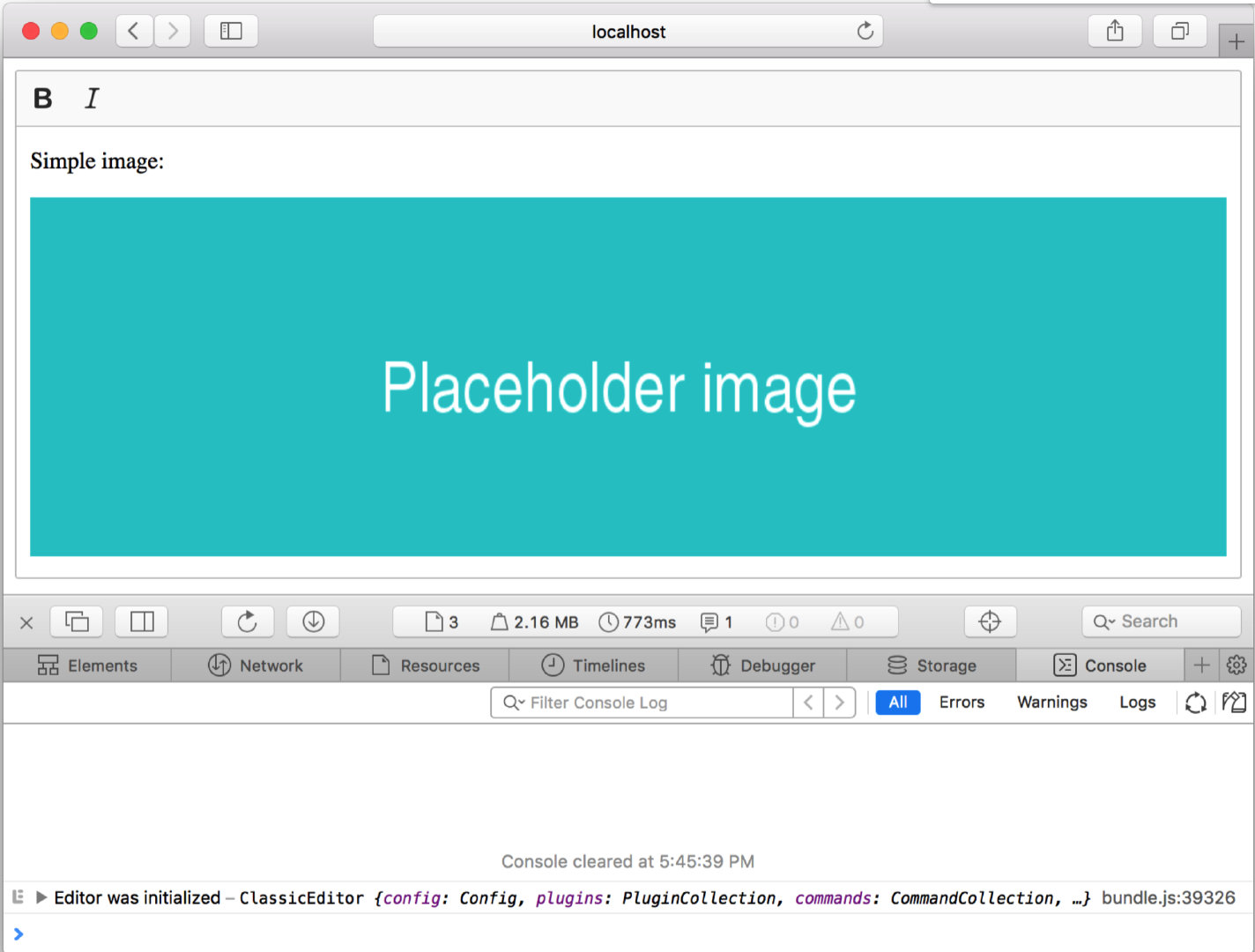
添加插件文件 insert-image.js
import Plugin from '@ckeditor/ckeditor5-core/src/plugin';
import imageIcon from '@ckeditor/ckeditor5-core/theme/icons/image.svg';
import ButtonView from '@ckeditor/ckeditor5-ui/src/button/buttonview';
export class InsertImage extends Plugin {
init () {
const editor = this.editor;
editor.ui.componentFactory.add('insertImage', locale => {
const view = new ButtonView(locale);
view.set({
label: 'Insert image',
icon: imageIcon,
tooltip: true
});
// Callback executed once the image is clicked.
view.on('execute', () => {
const imageURL = prompt('Image URL');
editor.model.change(writer => {
const imageElement = writer.createElement('imageBlock', {
src: imageURL
});
// Insert the image in the current selection location.
editor.model.insertContent(imageElement, editor.model.document.selection);
});
});
return view;
});
}
}
总结一下:
- 插件的作用、模板
- toolbar上添加按钮
插件的模板
import Plugin from "@ckeditor/ckeditor5-core/src/plugin";
export class PluginName extends Plugin {
init() {
const editor = this.editor;
console.log("plugin goes here.");
}
}
toolbar上添加按钮
ClassicEditor.create(document.querySelector("#editor"), {
plugins: [Essentials, Paragraph, Bold, Italic, Image, XxPlugin],
toolbar: ["bold", "italic", "xxFeature"],
});
import Plugin from "@ckeditor/ckeditor5-core/src/plugin";
export class XxPlugin extends Plugin {
init() {
const editor = this.editor;
editor.ui.componentFactory.add("xxFeature", (locale) => {
const view = new ButtonView(locale);
view.set({
label: "Insert image",
tooltip: true,
});
// Callback executed once the image is clicked.
view.on("execute", () => {
console.log("button click.");
});
return view;
});
}
}
最终的结构
.
├── app.js
├── dist
│ ├── bundle.js
│ └── bundle.js.map
├── index.html
├── insert-image.js
├── package-lock.json
├── package.json
└── webpack.config.js