Imooc - Python3 入门教程: Python变量和数据类型
第3章 Python变量和数据类型
3-1基本数据类型:
- 整数
- 浮点数(1.23 或者 科学计数:1.23x10^9)
- 科学计数: 1.23x10^9
- 存在 0.1 + 0.2 的问题
- 字符串('abc',"xyz")
- 布尔值(True/False)
- 空值(None)
Fei: 如何用Python实现字符串插值
# 保证顺序即可
print "Hi, I am {} and I am {} years old".format(age, name)
Hi, I am 26 and I am John years old
3-2 Python定义变量的方法
合法的:num, count, _none, min_value
- 变量名由大小写英文字母、数字和下划线_组成
- 变量不能用数字开头
- 变量尽量不要和Python关键字重合(比如前面学习过的:and、or、not,否则可能导致Python原有关键字发挥不出作用)
3-3 Python的整数与浮点数
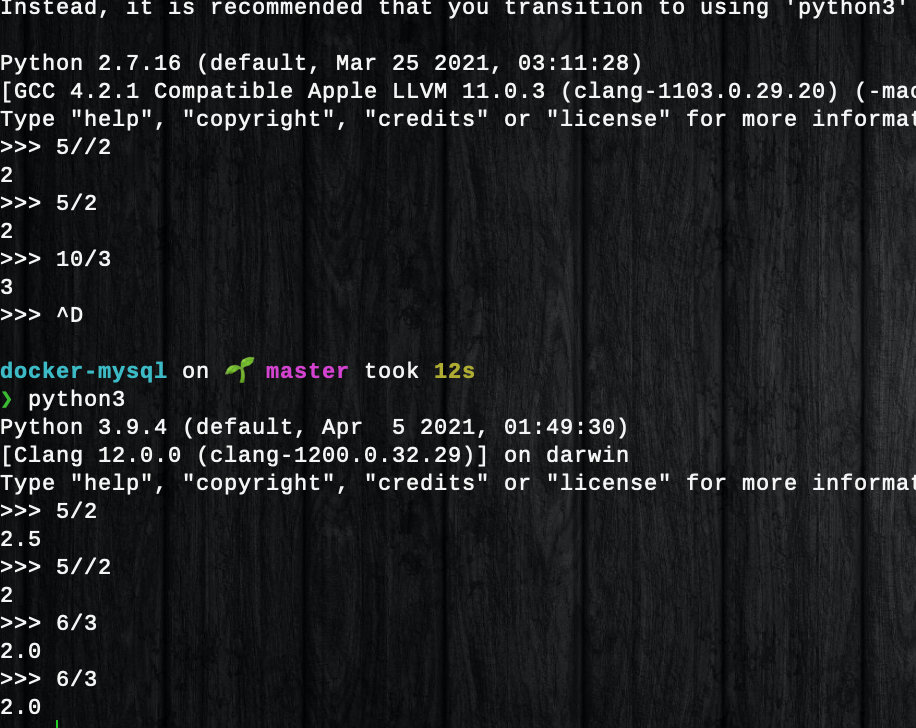
注意py2/py3在除法的区别
# python2
num1 = 10
num2 = 3
result = num1 / num2
print(result) # ==> 3
# python3
num1 = 10
num2 = 3
result = num1 / num2
print(result) # ==> 3.3333333333333335
# 使用round保留两位小数
round(num, 2) # ==> 3.33
地板除
10//4 # ==> 2
10//2.5 # ==> 4.0
10//3 # ==> 3
3-4 Python的布尔类型
与:a and b
或: a or b
非: not a
- 在计算a and b时,如果 a 是 False,则根据与运算法则,整个结果必定为 False,因此返回 a;如果 a 是 True,则整个计算结果必定取决与 b,因此返回 b。
- 在计算a or b时,如果 a 是 True,则根据或运算法则,整个计算结果必定为 True,因此返回 a;如果 a 是 False,则整个计算结果必定取决于 b,因此返回 b。
3-5 Python的字符串
常用的转义字符:
\n表示换行
\t 表示一个制表符
\\表示 \ 字符本身
3-6 Python中raw字符串与多行字符串
如果一个字符串包含很多需要转义的字符,对每一个字符都进行转义会很麻烦。为了避免这种情况,我们可以在字符串前面加个前缀r
,表示这是一个 raw
字符串,里面的字符就不需要转义了。例如:
# 第1种方式
r'\(~_~)/ \(~_~)/'
# 第2种方式
'''Line 1
Line 2
Line 3'''
3-7 Python的字符串format
# 顺序方式
template = 'Hello {0}, Hello {1}, Hello {2}, Hello {3}.'
# 有意义字符方式
# 指定{}的名字w,c,b,i
template = 'Hello {w}, Hello {c}, Hello {b}, Hello {i}.'
world = 'World'
china = 'China'
beijing = 'Beijing'
imooc = 'imooc'
# 指定名字对应的模板数据内容
result = template.format(w = world, c = china, b = beijing, i = imooc)
print(result) # ==> Hello World, Hello China, Hello Beijing, Hello imooc.
3-8 Python的字符串编码
s1 = '这是中文字符串'
s2 = 'this is an English string'
print(s1)
print(s2)
3-9 Python的字符串切片
其实就是 str.slice 方法
s = 'ABCDEFGHIJK'
abcd = s[0:4] # 取字符串s中的第一个字符到第五个字符,不包括第五个字符
print(abcd) # ==> ABCD
cdef = s[2:6] # 取字符串s中的第三个字符到第七个字符,不包括第七个字符
print(cdef) # ==> CDEF