Go语言学习: http 之 get 请求
基本的 GET 请求使用
安装
- 原生包,不用安装
main.go
最简单的无参数形式
get
请求
package main
import (
"io"
"log"
"net/http"
)
func main() {
apiurl := "https://httpbin.org/get"
response, _ := http.Get(apiurl)
body, _ := io.ReadAll(response.Body)
log.Printf("Response Body: %s", string(body))
}
带参数的
querystring
的情况安装包:
go get github.com/afeiship/nx
package main
import (
"io"
"log"
"net/http"
nx "github.com/afeiship/nx/lib"
)
func main() {
data := make(map[string]any)
data["name"] = "afei"
data["age"] = 25
apiurl := nx.Param(data, "https://httpbin.org/get")
response, _ := http.Get(apiurl)
body, _ := io.ReadAll(response.Body)
log.Printf("Response Body: %s", string(body))
}
HTTP 带 Headers
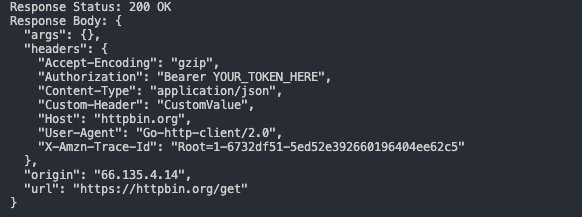
package main
import (
"fmt"
"io"
"net/http"
)
func main() {
client := &http.Client{}
// 创建请求,这里的 nil 不能为空
req, err := http.NewRequest("GET", "https://httpbin.org/get", nil)
if err != nil {
fmt.Println("Error creating request:", err)
return
}
// 添加 headers
req.Header.Add("Authorization", "Bearer YOUR_TOKEN_HERE")
req.Header.Add("Content-Type", "application/json")
req.Header.Add("Custom-Header", "CustomValue")
// 发送请求
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error making request:", err)
return
}
defer resp.Body.Close()
// 读取响应
body, err := io.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
fmt.Println("Response Status:", resp.Status)
fmt.Println("Response Body:", string(body))
}
使用 Fetch
包
package main
import (
"fmt"
"github.com/go-zoox/fetch"
)
func main() {
res, _ := fetch.Get("https://httpbin.org/get", &fetch.Config{
Headers: map[string]string{
"User-Agent": "fetch-example/1.0",
"X-Custom-Header": "custom-value",
},
})
fmt.Println(res)
}