rails: 多态关联(polymorphic)
关联中稍微高级一点的变体是多态关联。Rails 中的多态关联允许一个模型通过一个关联属于多个其他模型。当您有一个需要链接到不同类型的模型的模型时,这尤其有用。
例如,假设您有一个Picture
模型,它可以属于或Employee
,因为每个模型都可以有一个 个人Product
资料图片。以下是声明方式:
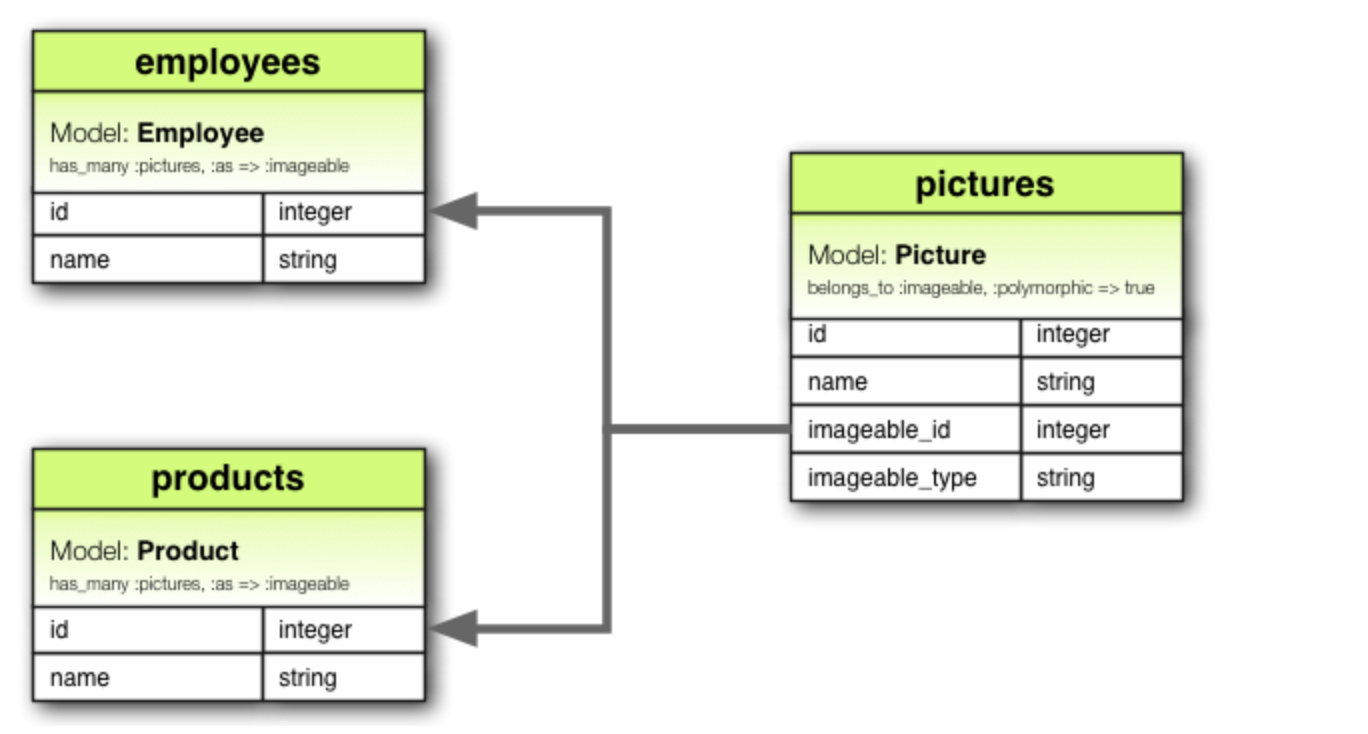
01 生成 Picture 模型
由于 Picture
模型需要与 Employee
和 Product
模型进行多态关联,我们需要在 Picture
模型中加入 imageable
相关字段。可以通过 rails generate model
命令来生成。
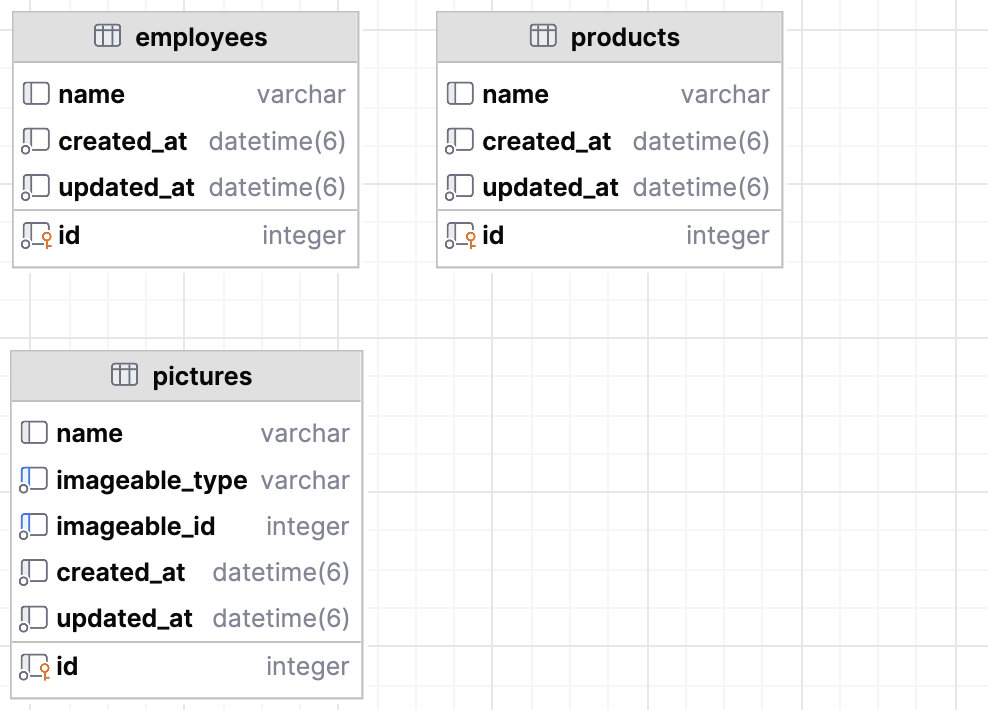
运行以下命令:
# 多态 model
rails g model Picture name imageable:references{polymorphic}
# 业务 model
rails g model Employee name
rails g model Product name
得到的
schema
imageable
polymorphic: true
class CreatePictures < ActiveRecord::Migration[8.0]
def change
create_table :pictures do |t|
t.references :imageable, polymorphic: true, null: false
t.timestamps
end
end
end
02 定义业务模型
生成模型后,你需要在各个模型文件中定义关联关系。
app/models/picture.rb
class Picture < ApplicationRecord
belongs_to :imageable, polymorphic: true
end
app/models/product.rb
class Product < ApplicationRecord
has_many :pictures, as: :imageable
end
app/models/product.rb
class Product < ApplicationRecord
has_many :pictures, as: :imageable
end
03 使用示例
# 创建 Employee 和 Product 对象
employee = Employee.create(name: "John Doe")
product = Product.create(name: "Laptop")
# 给 Employee 和 Product 添加图片
employee.pictures.create(name: "employee_pic.jpg")
product.pictures.create(name: "product_pic.jpg")
# 获取图片的关联对象
picture = Picture.first
picture.imageable # 这将返回关联的 Employee 或 Product 对象